A Comprehensive Guide to React Router DOM: Navigating Your React Applications
When it comes to building dynamic and interactive web applications with React, one essential aspect is navigation. Users need to move between different views or pages seamlessly, and that's where React Router DOM comes into play. In this guide, we'll explore React Router DOM in detail, providing clear and concise explanations to help you master this vital library for routing in your React applications.
What is React Router DOM?
React Router DOM is a library that enables navigation and routing in React applications. It offers a collection of components and a declarative way to define how your application's UI should change based on the current URL. With React Router DOM, you can create single-page applications (SPAs) with multiple "virtual" pages, allowing users to navigate through your application as if it were a traditional multi-page site.
Let's dive into the key concepts and components of React Router DOM:
Installation
To get started, you need to install React Router DOM in your project. You can do this using npm or yarn:
bash
npm install react-router-dom
or
yarn add react-router-dom
BrowserRouter
The `BrowserRouter` is a fundamental component that should wrap your entire application. It uses the HTML5 history API to keep the UI in sync with the current URL. Here's how you can set it up:
jsx
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Your application components */}
</Router>
);
}
Route
The `Route` component defines a mapping between a URL path and a React component. When the URL matches the path defined in a `Route`, the component is rendered. Here's an example:
jsx
import { Route } from 'react-router-dom';
function Home() {
return <h1>Home Page</h1>;
}
function About() {
return <h1>About Page</h1>;
}
function App() {
return (
<Router>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Router>
);
}
In this example, when the URL is `/`, the `Home` component is rendered, and when the URL is `/about`, the `About` component is rendered.
Link
The `Link` component provides a way to navigate between different pages in your application without triggering a full page reload. It generates an anchor (`<a>`) tag with an `href` that corresponds to the specified route.
jsx
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
);
}
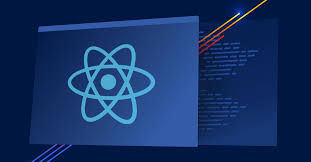
Nested Routes
You can also nest routes to create complex navigation hierarchies. This is useful for layouts with sidebars or multi-level menus. Here's an example:
jsx
import { Route, Switch } from 'react-router-dom';
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
{/* Nested Routes */}
<Switch>
<Route path="/dashboard/profile" component={Profile} />
<Route path="/dashboard/settings" component={Settings} />
</Switch>
</div>
);
}
function App() {
return (
<Router>
<Route path="/" exact component={Home} />
<Route path="/dashboard" component={Dashboard} />
</Router>
);
}
Route Parameters
Route parameters allow you to capture dynamic values from the URL and pass them as props to your components. For example:
jsx
import { Route } from 'react-router-dom';
function UserProfile() {
return (
<Route path="/user/:username">
{({ match }) => <h1>Hello, {match.params.username}!</h1>}
</Route>
);
}
In this case, if the URL is `/user/johndoe`, the component will display "Hello, johndoe!".
Putting It All Together
React Router DOM offers a powerful and flexible way to handle navigation in your React applications. By understanding the core components and concepts like `BrowserRouter`, `Route`, `Link`, and nested routes, you can build complex navigation structures with ease.
Remember to consult the official documentation for React Router DOM for more advanced features and techniques. With React Router DOM, you can create seamless, dynamic, and user-friendly single-page applications that provide a great user experience.
So, start routing your React applications with React Router DOM and take your web development to the next level!
Comments