Demystifying Redux: State Management for React Applications
State management is a fundamental aspect of building robust and maintainable React applications. As your application grows in complexity, managing state can become challenging. Redux is a popular library that addresses this challenge by providing a predictable and centralized way to manage state in your React applications. In this blog post, we'll explore what Redux is, why you should use it, and how it integrates with React using `react-redux`.
What is Redux?
Redux is a state management library for JavaScript applications, with a strong focus on React. It provides a predictable and centralized way to manage the state of your application. Redux follows the principles of a unidirectional data flow, which means that data in your application flows in a single direction, making it easier to understand and debug.
The core concepts of Redux include:
1.Store: The store is a JavaScript object that holds the state of your application. It's the single source of truth for your data.
2. Actions: Actions are plain JavaScript objects that describe what happened in your application. They are dispatched to the store and trigger state changes.
3. Reducers: Reducers are pure functions that specify how the application's state changes in response to actions. They take the current state and an action as input and return the new state.
4. Middleware: Middleware is used to handle asynchronous actions and side effects in Redux. It can be used to perform tasks like making API requests or logging.
Why Use Redux?
Now that we have a basic understanding of what Redux is, let's explore why you should consider using it in your React applications.
1.Centralized State Management: Redux provides a single source of truth for your application's state. This makes it easier to understand and maintain your application's data flow, especially in large and complex applications.
2. Predictable State Changes: Redux enforces a strict unidirectional data flow, which means that state changes are predictable and traceable. Actions are the only way to modify the state, making it easy to debug issues.
3. DevTools: Redux comes with powerful developer tools that allow you to inspect the state, actions, and even time-travel through your application's state changes for debugging purposes.
4. Reusable Code: Redux encourages the separation of state management logic from your UI components. This separation makes your code more modular and allows for easier code reuse.
5. Community and Ecosystem: Redux has a vibrant community and a vast ecosystem of packages and extensions, which can help you solve common state management challenges.
Integrating Redux with React using `react-redux`
To use Redux in your React application, you need to install both the `redux` and `react-redux` packages. `react-redux` provides a set of bindings for React, making it easier to connect your components to the Redux store.
Here's a simplified example of how to integrate Redux with React:
Step 1: Setting up the Redux Store
jsx
// store.js
import { createStore } from 'redux';
import rootReducer from './reducers'; // Your application's root reducer
const store = createStore(rootReducer);
export default store;
Step 2: Creating Actions and Reducers
Define actions and reducers to manage your application's state.
JSX
// actions.js
export const increment = () => ({ type: 'INCREMENT' });
export const decrement = () => ({ type: 'DECREMENT' });
jsx
// reducers.js
const initialState = { count: 0 };
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
export default rootReducer;
Step 3: Connecting Components
Use the `connect` function from `react-redux` to connect your React components to the Redux store.
JSX
// Counter.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './actions';
function Counter({ count, increment, decrement }) {
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = {
increment,
decrement,
};
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Step 4: Provider Component
Wrap your entire application with the `Provider` component from `react-redux` to make the Redux store accessible to your components.
jsx
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter';
function App() {
return (
<Provider store={store}>
<div>
<h1>Redux Counter App</h1>
<Counter />
</div>
</Provider>
);
}
export default App;
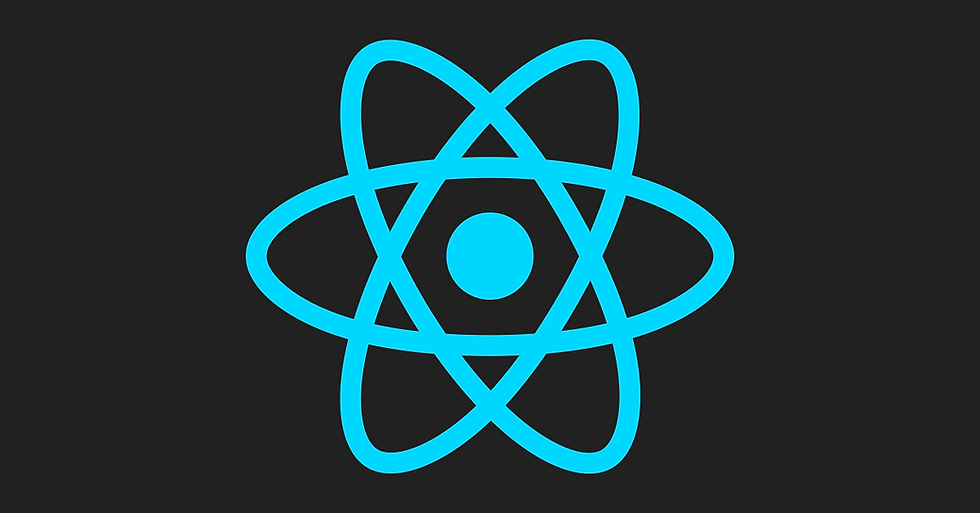
Conclusion
Redux is a powerful state management library that provides a predictable and centralized way to manage state in your React applications. While it adds some complexity to your code, especially for smaller applications, it becomes incredibly beneficial as your app grows in size and complexity. By using `react-redux` to connect your React components to the Redux store, you can take full advantage of Redux's features and benefits in your application development journey.
Comments