In today's web development landscape, making HTTP requests is an essential part of building modern web applications. Whether you need to fetch data from an API, submit form data to a server, or perform any other data exchange with a remote server, you'll likely encounter the need for a robust and versatile HTTP client. In this blog post, we will explore Axios, a popular JavaScript library for making HTTP requests, and learn how it simplifies the process of working with APIs and servers.
What is Axios?
Axios is a promise-based HTTP client for JavaScript that can be used both in the browser and Node.js environments. It provides a simple and elegant API for sending HTTP requests and handling responses. Axios is widely adopted in the web development community due to its ease of use, versatility, and excellent support for handling various types of requests, including GET, POST, PUT, DELETE, and more.
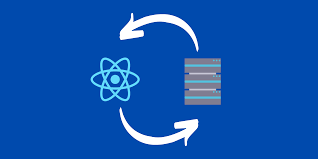
Installing Axios
To get started with Axios, you can add it to your project using npm or yarn:
bash
npm install Axios
or
yarn add Axios
Once Axios is installed, you can import it into your JavaScript files and start using it.
JavaScript
import axios from 'axios';
Making GET Requests
Let's begin by making a simple GET request to retrieve data from an API. Axios provides a straightforward syntax for this task:
JavaScript
axios.get('https://api.example.com/posts')
.then((response) => {
// Handle the successful response here
console.log(response.data);
})
.catch((error) => {
// Handle any errors here
console.error(error);
});
In this example, we use the `axios.get` method to send a GET request to the specified URL. We then use `.then` and `.catch` to handle the response or any potential errors.
Sending POST Requests
Sending POST requests with Axios is equally simple. Suppose you want to send data to a server to create a new resource, like adding a new post. Here's how you can do it:
javascript
const postData = {
title: 'New Post',
content: 'This is the content of the new post.',
};
axios.post('https://api.example.com/posts', postData)
.then((response) => {
// Handle the successful response here
console.log(response.data);
})
.catch((error) => {
// Handle any errors here
console.error(error);
});
Axios allows you to send data in the request body, making it perfect for sending form data, JSON payloads, or any other data format required by the server.
Handling Errors
Axios provides robust error handling through the use of `.catch` blocks. You can catch and handle various types of errors, such as network errors, invalid responses, or server errors. This ensures that your application remains stable and responsive even when unexpected issues occur during HTTP requests.
Interceptors and Global Configuration
Axios offers features like interceptors and global configuration, allowing you to customize request and response handling globally for your application. This can be helpful for tasks like adding authentication headers, error handling, or request logging.
Conclusion
Axios is a powerful and user-friendly HTTP client for JavaScript that simplifies the process of making HTTP requests in both browser and Node.js environments. Its intuitive API, error handling capabilities, and support for various request types make it a preferred choice for developers working with APIs and remote servers.
As you continue to explore web development, having a solid understanding of Axios will empower you to create dynamic and interactive web applications that seamlessly interact with remote data sources. So, go ahead and integrate Axios into your projects, and you'll find that handling HTTP requests has never been easier.
Comments