React Redux middleware is a critical part of the React Redux library, which is commonly used for managing the state of React applications. Middleware in Redux provides a way to extend the behavior of the Redux store's dispatch function, allowing you to intercept and modify actions before they reach the reducers or perform asynchronous tasks.
Here's how middleware works in React Redux:
1.Middleware Chain: Middleware is applied as a chain. When you dispatch an action, it passes through this chain of middleware functions before reaching the reducers.
2. Action Manipulation: Middleware can inspect, modify, or even block actions before they reach the reducers. This makes it useful for tasks like logging, authentication, or handling asynchronous operations.
3. Asynchronous Operations: Middleware is particularly valuable for handling asynchronous actions, like making API requests. It can delay the dispatch of an action until an asynchronous operation is completed and then dispatch a new action with the result.
4. Examples of Middleware: Popular middleware libraries for React Redux include Redux Thunk, Redux Saga, and Redux Promise. These libraries provide tools and patterns for handling asynchronous actions effectively.
Here's an example of how Redux Thunk middleware can be used to handle asynchronous actions:
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(thunk)
);
// Action creator with async logic using Redux Thunk
const fetchData = () => {
return (dispatch) => {
dispatch({ type: 'FETCH_DATA_REQUEST' });
// Perform an async operation, e.g., fetching data from an API
fetch('https://example.com/api/data')
.then((response) => response.json())
.then((data) => {
dispatch({ type: 'FETCH_DATA_SUCCESS', payload: data });
})
.catch((error) => {
dispatch({ type: 'FETCH_DATA_FAILURE', error: error.message });
});
};
};
// Dispatch an async action
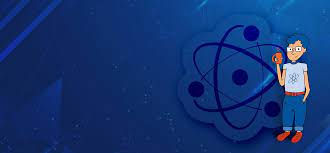
store.dispatch(fetchData());
In this example, Redux Thunk middleware allows you to return a function from an action creator, giving you access to the `dispatch` function to dispatch actions asynchronously. This middleware handles the execution of the function and dispatches the appropriate actions at the right time.
Middleware in React Redux enhances the flexibility and power of Redux, enabling you to manage complex state and asynchronous operations in your React applications more effectively. Different middleware libraries provide various approaches to handle asynchronous actions, so you can choose the one that best fits your project's needs.
Kommentare