Understanding the Internal Workings of React: A Deep Dive
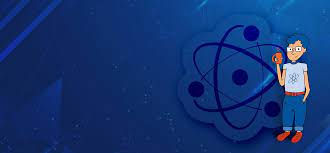
React has become a powerhouse in the world of web development. Its declarative approach to building user interfaces has won the hearts of developers around the globe. While many developers are comfortable using React to create stunning web applications, few understand its internal workings. In this blog post, we'll take a deep dive into the inner workings of React and demystify some of its core concepts.
What is React?
Before we dive into React's internals, let's briefly recap what React is. React is an open-source JavaScript library for building user interfaces, primarily for web applications. Developed by Facebook, it was released in 2013 and has since gained immense popularity. React is all about building reusable UI components and efficiently updating the user interface as the application's data changes.
The Virtual DOM
At the heart of React's efficiency lies the Virtual DOM. The Virtual DOM is a lightweight, in-memory representation of the actual DOM (Document Object Model). It serves as an intermediary between the application's data and the browser's rendering engine. Here's how it works:
1. Component Rendering: When a React component renders, it generates a Virtual DOM tree. This tree contains elements representing the component's structure.
2. Diffing Algorithm: When the application's data changes (e.g., due to user interactions), React creates a new Virtual DOM tree.
3. Reconciliation: React then compares the new Virtual DOM tree with the previous one, using a process called reconciliation. It identifies the differences, or "diffs," between the two trees.
4. Minimal Updates: Instead of directly updating the actual DOM for every change, React calculates the minimal set of updates needed to reflect the changes.
5. Efficient Rendering: Finally, React applies these updates to the real DOM, resulting in an efficient rendering process.
This Virtual DOM approach minimizes direct manipulation of the DOM, which can be slow and resource-intensive, leading to better performance.
Components and Props
React applications are built using components. Components are like building blocks that encapsulate a part of the UI's functionality and appearance. React components can be divided into two main types: functional components and class components.
Functional Components
Functional components are JavaScript functions that receive `props` (short for properties) as input and return JSX (JavaScript XML) as output. Props are used to pass data from a parent component to a child component. Here's a simple functional component:
jsx
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Class Components
Class components are ES6 classes that extend the `React.Component` class. They have a `render()` method that returns JSX. Class components also support state management, allowing for more complex behavior. Here's an example:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>Increment</button>
</div>
);
}
}
State Management
State management is a crucial part of React's internal workings. State represents the data that can change over time within a component. In class components, state is managed using the `this.state` object. When state changes, React re-renders the component, updating the Virtual DOM and, consequently, the real DOM as well.
In functional components, the introduction of the `useState` hook in React's newer versions allows functional components to manage state, making them more powerful and versatile.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
The Component Lifecycle
React components go through a lifecycle from birth to death, and developers can hook into different stages of this lifecycle to perform actions. Key lifecycle methods include:
componentDidMount: Called after a component is rendered to the DOM. Useful for performing initial setup, like fetching data from an API.
componentDidUpdate: Called after a component's state or props change and it re-renders.
componentWillUnmoun: Called right before a component is removed from the DOM. Useful for cleaning up resources, like event listeners.
React also introduced the concept of "side effects" using hooks like `useEffect` in functional components. This hook allows developers to manage the component's lifecycle-related tasks.
Conclusion
React's internal workings may seem complex at first glance, but understanding its core concepts, such as the Virtual DOM, components, state management, and the component lifecycle, is essential for becoming a proficient React developer. These foundations empower developers to build efficient and maintainable web applications with ease. By grasping these concepts, you'll be well on your way to mastering React and creating captivating user interfaces.
留言