React, a JavaScript library for building user interfaces, has revolutionized the way we develop web applications. One of its key features is the useState method, which allows you to manage state within your components. State management is crucial in React because it enables your components to maintain and display dynamic data. In this blog post, we'll dive deep into the useState method, exploring its purpose, usage, and best practices.
## What is useState?
In React, state is a fundamental concept that represents the data a component needs to render and manage over time. The useState method is a built-in hook that provides a way to declare and manage state in functional components. Before the introduction of hooks in React, state management was primarily done using class components and the `setState` method.
The useState hook simplifies state management by allowing you to add state to functional components without the need for a class component. It's a simple yet powerful tool that opens up the world of state management to functional programming enthusiasts.
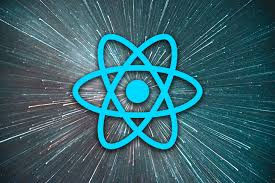
Basic Usage
To use the useState hook, you first need to import it from the React library:
JSX
import React, { useState } from 'react';
Now, let's create a simple functional component with state using the useState hook:
JSX
function Counter() {
// Declare a state variable 'count' with an initial value of 0
const [count, setCount] = useState(0);
// Event handler to increment the count
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
In this example, we declare a state variable `count` and its corresponding updater function `setCount`. The `useState` hook accepts the initial state as an argument, which, in this case, is set to 0. We display the value of `count` in the component's render method and provide a button that, when clicked, triggers the `increment` function to update the state.
Updating State
When you call the `setCount` function, it not only updates the state but also re-renders the component with the new state. React efficiently manages this process, ensuring that your UI reflects the latest state. It's essential to understand that state updates are asynchronous, and React batches them for performance reasons.
Here's another example that illustrates how to update state based on the previous state:
JSX
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount((prevCount) => prevCount + 1);
};
const decrement = () => {
setCount((prevCount) => prevCount - 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
In this example, we use a functional update to ensure that state updates are based on the previous state. This is particularly important when multiple updates can happen in quick succession.
Best Practices
To make the most of the `useState` hook in React, consider the following best practices:
1.Declare Multiple State Variables: It's common to have multiple pieces of state in a component. Declare them separately using the `useState` hook for clarity and maintainability.
2Functional Updates: Use functional updates when updating state based on the previous state to avoid issues with asynchronous updates.
3. Extracting State Logic: If you find yourself reusing state logic across multiple components, consider creating custom hooks to encapsulate that logic for better code reuse.
4. Avoid Nested Hooks: Do not use hooks inside loops, conditions, or nested functions. Hooks should always be used at the top level of your functional components.
5. Keep Components Small: Break down your components into smaller, manageable pieces. Smaller components are easier to understand and maintain.
Conclusion
The `useState` hook is a fundamental building block for managing state in React functional components. It simplifies state management, making it accessible to developers who prefer functional programming patterns. By following best practices and understanding its usage, you can harness the power of state in React and create dynamic and interactive user interfaces. So, go ahead and start using the `useState` hook in your React applications to build amazing user experiences.
Comments